How to send a whatsapp message using Node.js and Twilio API
- aleksvp
- Nov 25, 2020
- 1 min read
Full code: https://github.com/aleksvp2017/nodewhatsapp
Using:
Node.js;
Twilio;
Visual Studio Code.
First things first. There are two ways of sending messages through whatsapp:
Create a commercial account to use WhatsApp Business API directly. But it is not easy, there is an approval and business verification process. It is clearly directed to big companies like Booking.com, Uber, Ifood, etc. Anyway, you can try it here: https://www.facebook.com/business/m/whatsapp/business-api;
Use a integration partner, that is a company that already has the relationship with whatsapp and offers an API. This is the option I'm going to show, in particular, using Twilio API.
The first step is to creat an account on https://www.twilio.com/try-twilio. It is completely free and you got U$15 bonus to use in production mode.
The next step is to add a verified number (https://www.twilio.com/console/phone-numbers/verified).
Then you are ready to go.
The code is very simple actually. Besides the express setup and some simple checks, to actually send a message, all you need is to call a simples function. The full code is this:
var express = require('express') var app = express() var cors = require('cors') app.use(cors()) app.get('/', function (req, res) { const client = require('twilio')() var recipient = req.query.number var msg = req.query.msg if (!recipient || !msg){ res.send('Please send query parameters msg and number. Ex: http://localhost:8080/?msg=test&number=111111111') return } client.messages.create({ from: 'whatsapp:+14155238886', body: msg, to: 'whatsapp:'+recipient }).then(message => { console.log('Message sent: ', message.sid) res.send('Message sent: ' + message.sid); }).catch(error => { console.log(error) res.send('Message not send. Error: ' + error); }) }) app.listen('8080', () => { console.log('Server up and listening at 8080')})
Very easy, right?
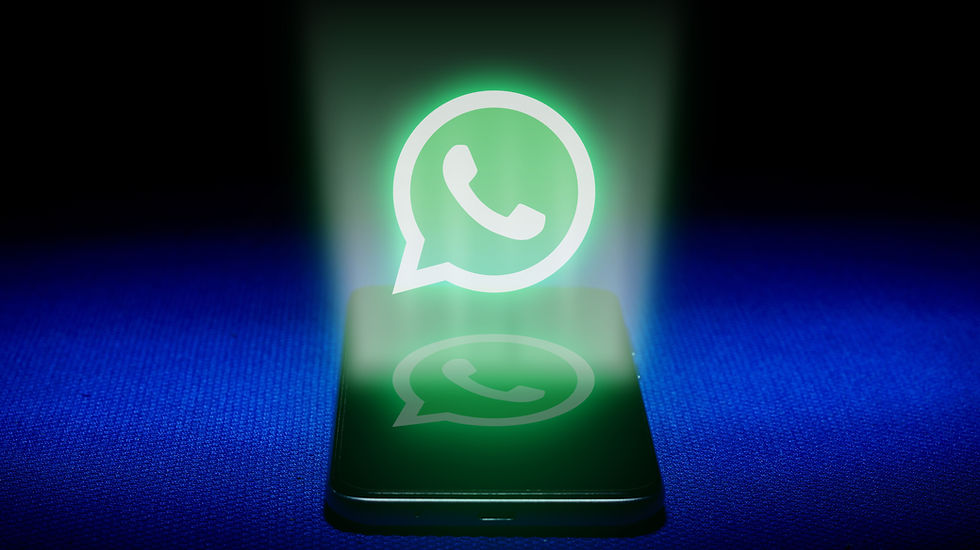
Comments